The State of Vue.js Report 2025 is now available! Case studies, key trends and community insights.
Table of Contents
Please note that Vue Native is no longer maintained and has been deprecated, so some information from this post is out of date.
For more up-to-date information on Vue, please visit our Vue Development Guide:
Adding Vue.js to Your Technology Stack
The mobile app development world is saturated with numerous frameworks. You have React Native, Flutter, NativeScript, Vue Native, and many, many more. All these tools offer a great development experience conducive to building excellent apps.
:quality(90))
Still, it’s Vue Native, a relative newcomer, that has sparked over 2,500 projects within only days of its GitHub premier. So what does this JavaScript mobile app development framework have to offer that it generates such considerable buzz? Let’s find out.
What is Vue Native and how it came to be?
Vue Native is a JavaScript framework for building native mobile apps using Vue.js. Vue Native transpiles files to React Native, which results in native apps for iOS and Android.
Choosing Vue.js, everything you need to know.
In essence, a Vue Native app is a wrapper around React Native APIs. Vue Native brings together React Native and Vue.js, letting developers take the best of each and build apps with rich UI.
Interestingly, Vue Native started out as a side project named react-vue, built to run Vue inside React and React Native. The initial project was developed by SmallComfort and later forked by GeekyAnts, who expanded the project into Vue Native as it is today.
GeekyAnts appreciated Vue.js’ support for templating, styling, state management in Vuex, and router and wanted to bring the same into Vue Native. The result is a cross-platform native mobile development framework for building lightweight apps.
In terms of popularity, Vue Native is still to appear on developer surveys. This can be ascribed to its relatively young age — Vue Native was officially released in 2018.
Vue Native features and how they function?Two-way binding
Vue Native supports two-way binding with a v-model directive. Two-way binding instantly propagates any data changes to the output, eliminating the need to write additional logic.
Changes in the model are instantly reflected in the UI. When the UI is updated, the changes are reflected in the model. This approach reduces the time necessary for a changed UI element to render.
Declarative rendering
Declarative rendering is at the core of reactivity in Vue Native — the data and DOMs are connected, meaning when variables are set to different values, they render immediately.
Reusable UI components
Vue Native, just like Vue.js, lets devs build reusable UI elements.
Component reusability is one of the best ways to achieve reduced development time. You can freely adapt individual components to work across different platforms (Android, iOS, and web).
Reusable UI components save time needed to build templates and designs, giving developers more time to implement business logic.
Also, because Vue Native is a wrapper around React Native, you can freely use all React Native components in an application, and the thing with React Native is that it’s rich in third-party libraries and support.
Vue Native pros and cons
Pros
Syntactic sugar
Vue Native is lauded by developers as having a clear and brief code that’s easy to read, write, and grasp — i.e, syntactic sugar.
Syntactic sugar means that a single command is expressed differently. The changed expression is meant to make the command simpler and more efficient. In JavaScript, frameworks that sweeten up the code strive to make it more dynamic and easy for newcomers to learn.
With too much syntactic sugar, however, the code becomes illegible instead of easier to learn and understand. Vue Native strikes a fine balance between being efficiently sweet to express and not overcomplicated.
Code written in Vue Native is concise and efficient — needless to say, a lot can be achieved with fewer lines of code.
Vue ecosystem
Since being released in 2014, Vue.js has built a robust library of tools. When using Vue Native, you gain access to all the good things lurking inside.
Within that ecosystem you’ve got:
- Vue CLI (ver. 4)
- Vuex
- Vue Native Router
- Vue Test Utils
...and plenty of solutions developed specifically to solve pressing developer problems. In short, you can pick recommended solutions without having to browse through piles of plugins in search of quality ones that are stable.
Moreover, Vue CLI in its third version gives you rapid prototyping, a rich collection of plugins, interactive project scaffolding. GUI (graphic user interface) support that further speeds up development.
Gentle learning curve
Vue Native lets developers familiar with JavaScript, CSS, and HTML build native mobile apps without expertise in iOS or Android.
Great documentation
Vue Native has in-depth documentation for reference—developers can easily learn how to build apps using Vue Native. Every Vue Native functionality is explained, descriptions suitable for beginner and experienced devs.
If you’re a seasoned dev, in the official documentation, you can appreciate further nuances regarding specific functionalities and detailed descriptions of how the framework itself operates.
Cons
Being a relatively new framework, Vue Native has some specific drawbacks:
- More advanced, in-depth information is missing in Vue Native documentation - you need to dive into React Native documentation to find answers.
- Although Vue Native allows you to use libraries designed for React Native, sometimes you will need to know React to adapt them to the needs of the Vue Native project.
Additionally, since Vue Native is a wrapper around React Native APIs, they share all the cons. Namely:
- It’s not a fully cross-platform, single-codebase approach.
- It lacks some custom, platform-specific modules and you may need expertise from a native developer to create them.
- The navigation is not as smooth and cross-platform development might cause performance and device-related app issues.
- It's not the best choice for apps that include games or heavy animations.
But to get a better feel of how working with Vue Native looks like and to be able to evaluate it properly, let’s dive into an (almost) hands-on experience.
(And if you’ve had enough of reading for now and would prefer a video, check out this example)
Source: Andre Madarang’s Youtube channel
Vue Native app tutorial
Setting up a Vue Native app
To start working with Vue Native, you’ll need a globally installed:
- node
- npm
There are two main ways to setup React Native application and (by extension) a Vue Native one.
If you’re not familiar with mobile development, the easiest way would be to use Expo CLI. It is a framework and a platform built around React Native focused on simplifying development, build, and deployment processes of mobile applications.
More advanced users may want to use React Native CLI. This approach requires installed Xcode or Android Studio to get started and is a bit more difficult to set up but also gives you more freedom.
Whichever you choose, the easiest way to get started with Vue Native is with Vue Native CLI. This tool will help you initialize a project. Let’s do it with Expo this time.
First, install Vue Native CLI and Expo CLI:
npm install --global vue-native-cli
npm install --global expo-cli
Create a new Vue Native project:
vue-native init <project-name>
That was easy, wasn’t it?
Now let’s start the local development server of Expo CLI:
cd <project-name>
npm run start
You should be able to see a browser window with Expo Developer Tools. To open our app on your smartphone, make sure that you have the Expo app installed on your device. Scan QR code from Expo Developer Tools with it and wait until the application is loaded.
Your app should now be visible on your device. More importantly, if you start playing around with a code, you’ll notice that your changes are immediately visible.
Creating a simple app
Lets create a simple app that shows a list of Vue Native contributors with their login, avatar, and number of contributions. We are going to fetch the list from GitHub REST API.
Start with a simple App.vue
file:
<!-- App.vue -->
<template>
<view :style="{ paddingVertical: 40 }">
<text class="header">Vue Native contributors</text>
<!-- List should goes here -->
</view>
</template>
<style>
.header {
font-size: 24px;
margin-bottom: 20px;
text-align: center;
}
</style>
To access data from REST API we can use Fetch API in the same way as in web application. According to GitHub Docs GET /repos/{owner}/{repo}/contributors
responds with a list of contributors to the specified repository. Let’s fetch this list when the application is created and save it in component data. We need to add <script>
block to our component:
<!-- App.vue -->
...
<script>
export default {
data() {
return {
users: []
}
},
async created() {
const url = 'https://api.github.com/repos/GeekyAnts/vue-native-core/contributors'
this.users = await fetch(url)
.then(res => res.json())
}
}
</script>
...
We can also show usernames of fetched contributors:
<!-- App.vue -->
<template>
<view :style="{ paddingVertical: 40 }">
<text class="header">Vue Native contributors</text>
<text
v-for="user in users"
:key="user.id"
>
{% raw %}{{ user.login }}{% endraw %}
</text>
</view>
</template>
...

It looks like it works! Now let’s make it pretty. We will create a new component called GithubUser
to show a single user.
<!-- components/GithubUser.vue -->
<template>
<view class="github-user">
<image
class="github-user__image"
:source="{ uri: user.avatar_url }"
/>
{% raw %}<text>{{ user.login }}</text>
<text>{{ user.contributions }} contributions</text>{% endraw %}
</view>
</template>
<script>
export default {
props: {
user: Object
}
}
</script>
Lets style it to center image and username within a single item. To achieve it I added align-items: center
style for the wrapper. I also specified dimensions of an image and also made it round:
<!-- components/GithubUser.vue -->
...
<style>
.github-user {
padding: 16px;
align-items: center;
}
.github-user__image {
width: 50px;
height: 50px;
border-radius: 25px;
margin-bottom: 5px;
}
</style>
Let's use this component:
<!-- App.vue -->
<template>
<view :style="{ paddingVertical: 40 }">
<text class="header">Vue Native contributors</text>
<github-user
v-for="user in users"
:key="user.id"
:user="user"
/>
</view>
</template>
<script>
import GithubUser from './components/GithubUser.vue'
export default {
components: {
GithubUser
},
// ...
}
</script>
...

We are almost there! After running this application you will notice that list is overflows main view and you are not able to scroll the list. To make a view scrollable you'll need a special Vue Native component ScrollView. Let's replace our main wrapper with this component:
<!-- App.vue -->
<template>
<scroll-view :content-container-style="{ paddingVertical: 40 }">
<text class="header">Vue Native contributors</text>
<github-user
v-for="user in users"
:key="user.id"
:user="user"
/>
</scroll-view>
</template>
...
And that's it!

Full example:
<!-- App.vue -->
<template>
<scroll-view :content-container-style="{ paddingVertical: 40 }">
<text class="header">Vue Native contributors</text>
<github-user
v-for="user in users"
:key="user.id"
:user="user"
/>
</scroll-view>
</template>
<script>
import GithubUser from './components/GithubUser.vue'
export default {
components: {
GithubUser
},
data() {
return {
users: []
}
},
async created() {
const url = 'https://api.github.com/repos/GeekyAnts/vue-native-core/contributors'
this.users = await fetch(url)
.then(res => res.json())
}
}
</script>
<style>
.header {
font-size: 24px;
margin-bottom: 20px;
text-align: center;
}
</style>
and GithubUser
component:
<!-- components/GithubUser.vue -->
<template>
<view class="github-user">
<image
class="github-user__image"
:source="{ uri: user.avatar_url }"
/>
{% raw %}<text>{{ user.login }}</text>
<text>{{ user.contributions }} contributions</text>{% endraw %}
</view>
</template>
<script>
export default {
props: {
user: Object
}
}
</script>
<style>
.github-user {
padding: 16px;
align-items: center;
}
.github-user__image {
width: 50px;
height: 50px;
border-radius: 25px;
margin-bottom: 5px;
}
</style>
Styling a text input in Vue Native
Styling Vue Native component can be done in two ways:
- you can use inline styles
- you can define your stylesheet in
<style>
block
Styling Vue Native components is similar to styling Vue components for web application. Let’s compare both approaches and style text input with them. We will add some rounded border and specify the size of the input.
Here’s how it looks like with inline styles:
<template>
<text-input
:style="{
height: 40,
width: 200,
borderWidth: 1,
borderColor: 'gray',
borderRadius: 8,
padding: 8
}"
placeholder="Type a message..."
/>
</template>
And here the styles are moved to <style>
block:
<template>
<text-input
class="my-text-input"
placeholder="Type a message..."
/>
</template>
<style>
.my-text-input {
height: 40px;
width: 200px;
border-width: 1px;
border-color: gray;
border-radius: 8px;
padding: 8px;
}
</style>
Screenshots:
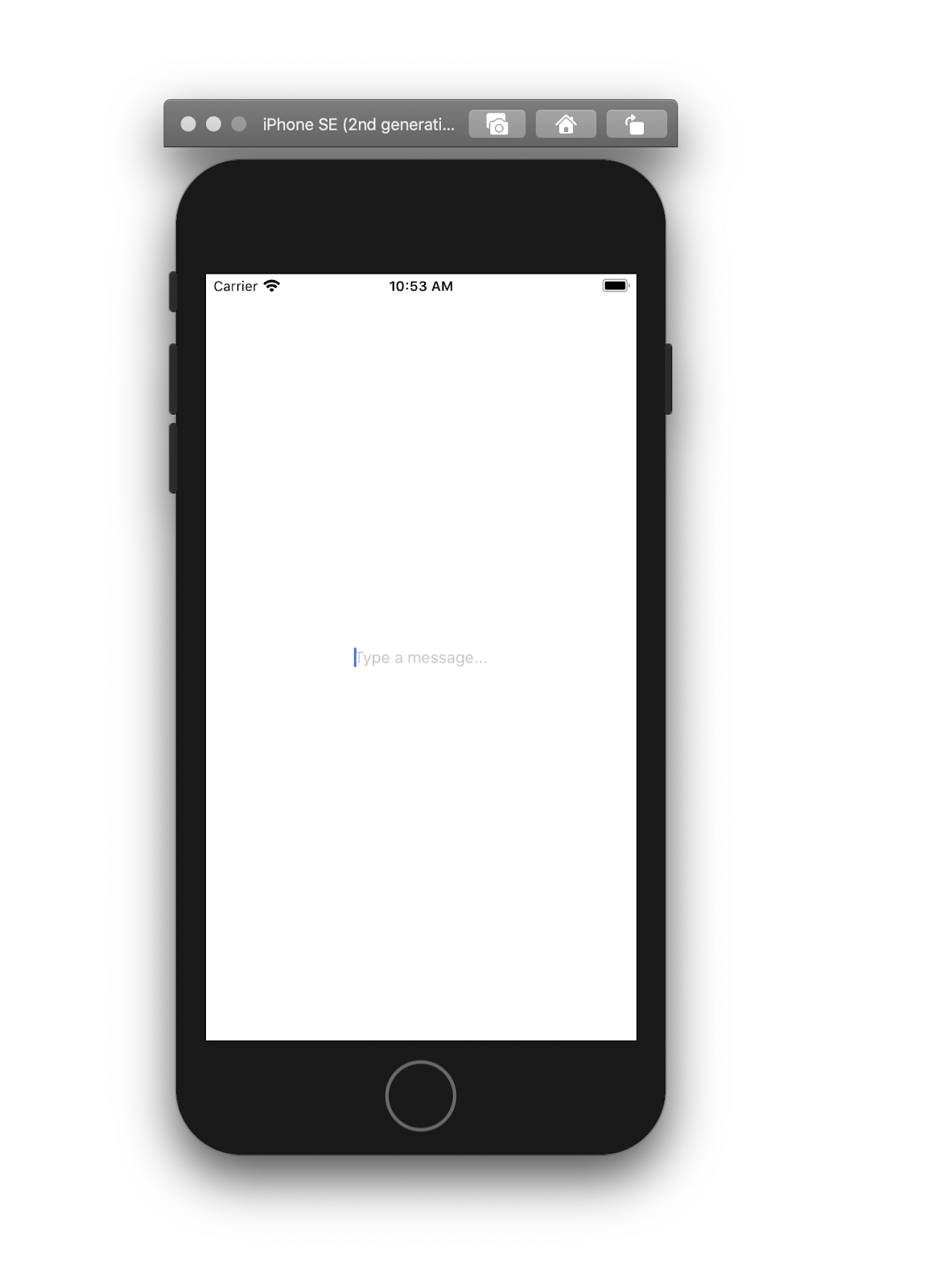
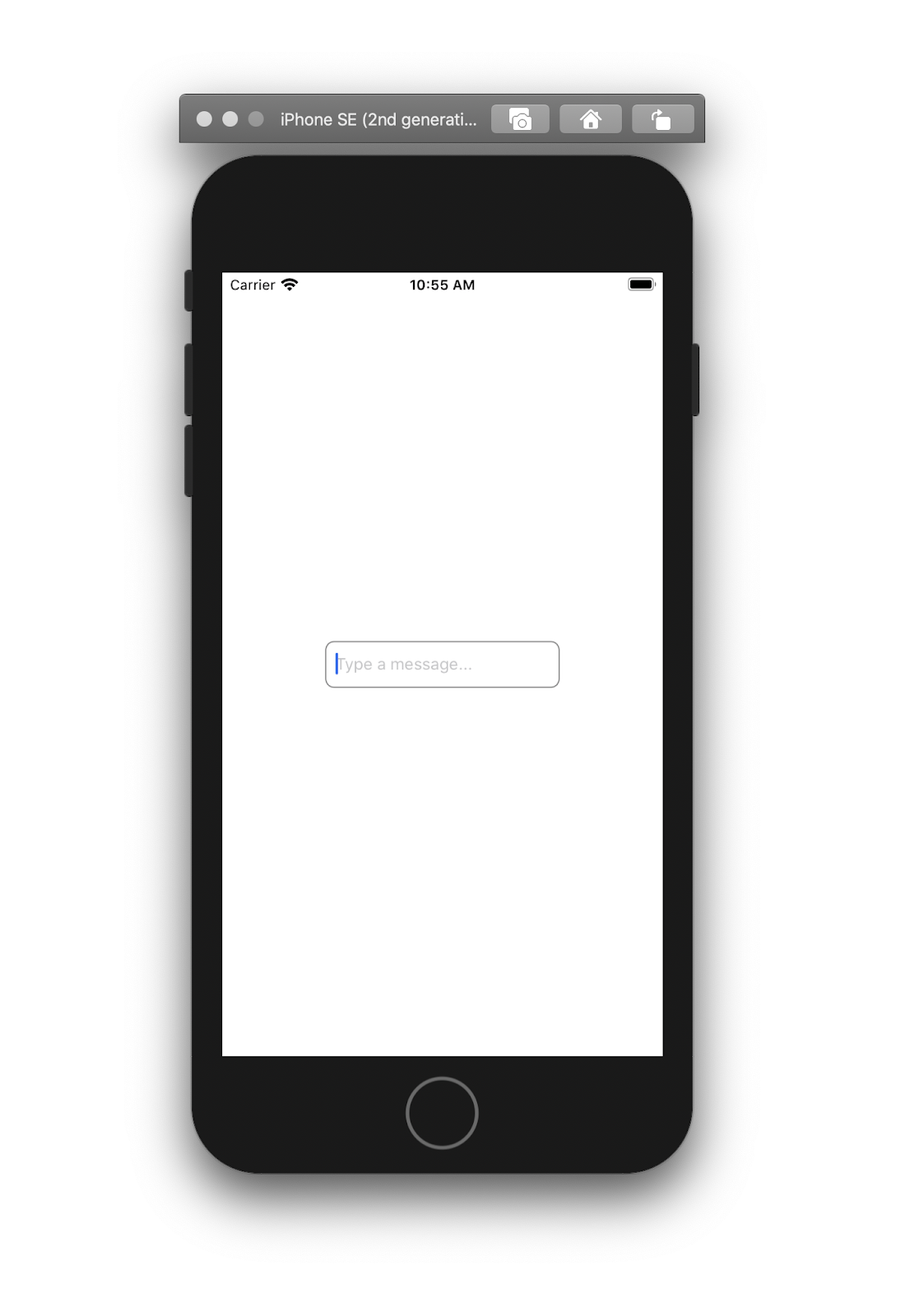
Who should use Vue Native?
The choice between Vue Native and other mobile development frameworks out there pretty much depends on your proficiency and curiosity.
If you’re familiar with Vue.js, working in Vue Native will be easier — there are a lot of similarities between the two. Besides, adding Vue Native to a Vue.js web app will be a nice tech stack extension for the mobile equivalent of your app.
However, if you’re more of a React Native person, switching to Vue Native could be a rewarding experience — a means to satisfy your developer’s curiosity. But work-wise, getting to know how to build apps in Vue Native could be inefficient in terms of time. After all, with both these frameworks, you’ll build an app of the same quality.
If you're thinking about adding Vue.js to your technology stack, download our comprehensive guide with all the insights, data, and case studies about Vue.js.
Is Vue Native on track to conquer the landscape of mobile app development frameworks?
In the sea of cross-platform mobile development frameworks, Vue Native combines the perks of two powerful tools: React Native and Vue.js. Both these tools are wildly popular among developers and many globally recognized companies have them in their stacks.
While it’s arguable whether Vue Native is better than other frameworks out there, it does offer developers a plethora of benefits that make development faster, easier, and more efficient. As Vue Native reaches adolescence, we can expect an increased adoption and a maturing of this framework that will doubtless be represented in favorable developer survey scores.
:quality(90))